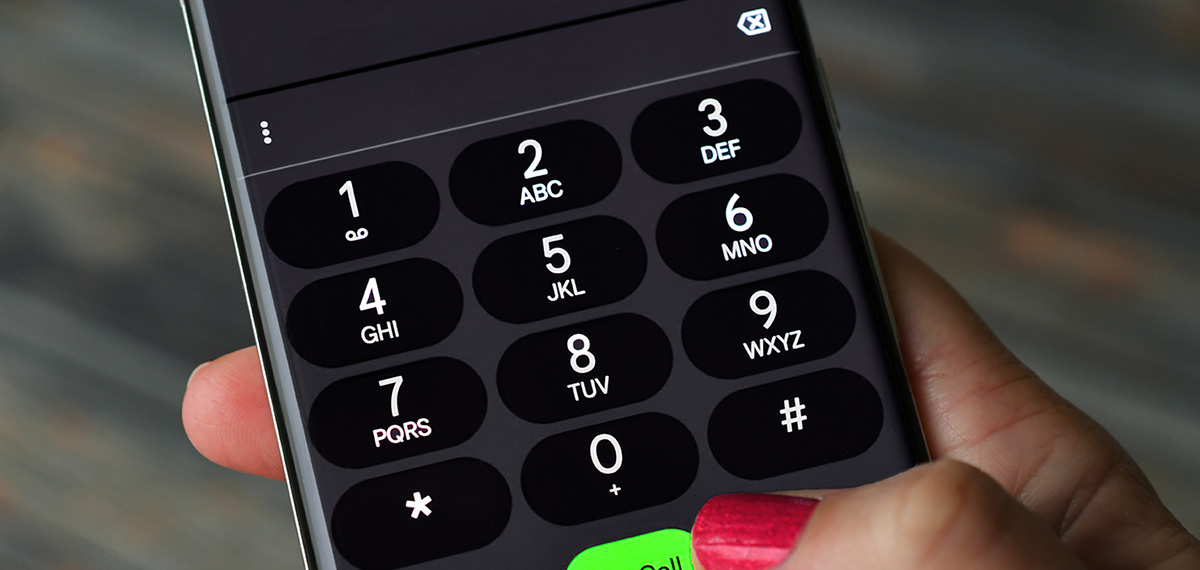
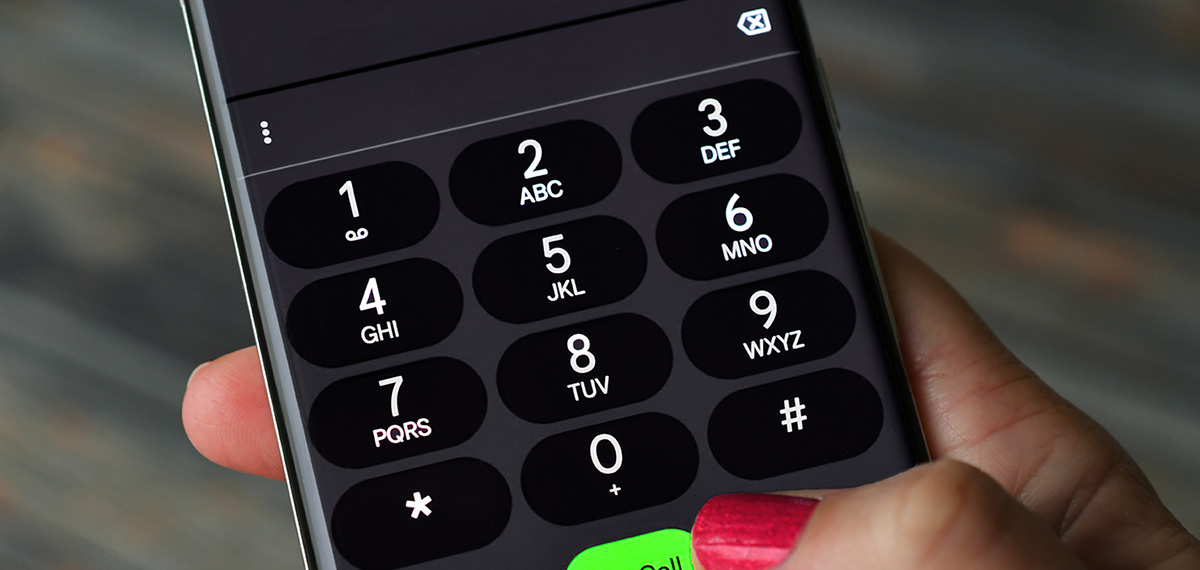
findTextonym(keys: string[]) {
if (this.inputCount == 0) {
this.inputDrafts = [...keys];
} else {
let extend = [];
for (let d = 0; d < this.inputDrafts.length; d++) {
for (let key of keys) {
const appended = `${this.inputDrafts[d]}${key}`;
extend.push(appended);
}
}
this.inputDrafts = [...this.inputDrafts, ...extend];
}
const inputDraftFiltered = this.inputDrafts.filter((word) => {
return word.length >= this.inputCount;
});
console.log('INPUT: ', this.inputDrafts.length);
console.log('INPUT FILTERED: ', inputDraftFiltered.length);
const matches = this.words.filter((word) => {
return (
inputDraftFiltered.includes(String(word).toUpperCase()) &&
word.length == this.inputCount + 1
);
});
if (Object.keys(this.dictByChar).length > 0) {
const firstKey: KeypadItem | undefined = this.keypadItems.find((item) => {
return item.keys.includes(this.inputDrafts[0]);
});
let filteredByLetters: string[] = [];
firstKey?.keys.map((key) => {
filteredByLetters = [
...filteredByLetters,
...this.dictByChar[String(key).toLowerCase()],
];
});
const matchByLetters: string[] = filteredByLetters.filter((word) => {
return (
inputDraftFiltered.includes(String(word).toUpperCase()) &&
word.length == this.inputCount + 1
);
});
this.matches = [...matches, ...matchByLetters];
} else {
this.matches = [...matches];
}
}